I am confused what the width of the slider widget is: I have a stack and added an align widget that contains a slider.
Code:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
#override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Stack(
children: [
Align(
alignment: Alignment.bottomCenter,
child: OptionsBar(),
),
],
),
),
);
}
}
class OptionsBar extends StatefulWidget {
const OptionsBar({Key? key}) : super(key: key);
#override
_OptionsBarState createState() => _OptionsBarState();
}
class _OptionsBarState extends State<OptionsBar> {
double progress = 0.0;
#override
Widget build(BuildContext context) {
return Container(
margin: EdgeInsets.all(10),
child: Row(
children: [
Slider(
value: progress,
onChanged: (double newValue) => setState(() => progress = newValue),
)
],
),
);
}
}
The code works but places the slider in the middle of the screen. However, if I give the widget that contains the slider a height than the bar remains at the bottom. So, does that mean that a slider takes up the entire height that it can get? Seems a little bit strange or am I getting something wrong?
You have set alignment to bottom that's why when you set height of widget it remains on the bottom. Try to set height to the widget that containing slider and change alignment.
Related
I try to make a list of widget. It look like this:
I know of no such thing as Constraint Layout in flutter. But I need something to position my arrow icon in a fixed position on the right. To put it simple, this is my widget code:
Row(
children:[
SizedBox(),
Column(),//this is all the item on the left
Spacer(),
Expanded(// this is the heart and arrow button
child: Column()
)
]
)
I notice that if my column on the left get too wide, my arrow and heart icon is shifted out of line.
How to put my icon in fixed position to the right?
here try this, You have to wrap middle column with expanded so it will take the maximum space available
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
/// This is the main application widget.
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Code Sample';
#override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: const Center(
child: MyStatefulWidget(),
),
),
);
}
}
/// This is the stateful widget that the main application instantiates.
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({Key? key}) : super(key: key);
#override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
/// This is the private State class that goes with MyStatefulWidget.
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool isChecked = false;
#override
Widget build(BuildContext context) {
return ListView.builder(
itemCount: 10,
itemBuilder: (_, index) => Container(
padding: EdgeInsets.all(15),
margin: EdgeInsets.symmetric(vertical: 5),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(10),border: Border.all(width: 1.5)),
child: Row(
children: [
Container(
width: 25,
height: 40,
color: Colors.black,
),
Expanded(
child: Column(children: [
//put your children here
]),
),
//this will be always on right
Column(
children: [
Icon(Icons.heart_broken),
Icon(Icons.chevron_right),
],
)
],
),
),
);
}
}
My Flutter desktop web app has complex ui that would be too tricky to make responsive. So instead I want to put it in a FittedBox that will simply shrink the whole app if the user makes the browser window smaller.
class CustomPage extends StatelessWidget {
final Widget child;
const CustomPage({
Key? key,
required this.child,
}) : super(key: key);
#override
Widget build(BuildContext context) {
return Scaffold(
body: GetPlatform.isDesktop
? FittedBox(
child: SizedBox(
width: Get.width,
height: Get.height,
child: Center(child: child),
),
)
: Text('Sorry! This was only meant for desktop.'),
);
}
}
But Get.width and WidgetsBinding.instance.window.physicalSize.width only get the initial window size. So if the app is started in a full screen browser then this works great, but if the app is started in a small browser it doesn't work. MediaQuery.of(context).size.width only gets the current screen size.
Is there a way of getting a desktop physical screen size?
you need to import
import 'dart:html';
then window.screen?.width and window.screen?.height
will give you physycal screen size
import 'dart:html';
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
#override
Widget build(BuildContext context) => MaterialApp(debugShowCheckedModeBanner: false, home: Home());
}
class Home extends StatelessWidget {
const Home({Key? key}) : super(key: key);
#override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(),
body: LayoutBuilder(builder: (context, c) {
return Center(
child: Text('${window.screen?.width} ${window.screen?.height}'),
);
}),
);
}
}
The code snippet below is how I generate my page. I detect the screenWidth and screenHeight and then use these values to detect if the page should be displayed in portrait or landscape.
The two factions return data type of Scaffold and the appropriate layout is rendered depending on screen orientation.
class UserInstructions extends StatefulWidget {
const UserInstructions({Key? key}) : super(key: key);
#override
_UserInstructionsState createState() => _UserInstructionsState();
}//***************** class UserInstructions ends **************************
class _UserInstructionsState extends State<UserInstructions> {
#override
Widget build(BuildContext context) {
double screenWidth = (MediaQuery.of(context).size.width) ;
double screenHeight = (MediaQuery.of(context).size.height);
if (screenWidth < screenHeight) { // we are in portrait mode
return portraitLayout();
}
else {//( screenWidth > screenHeight) we are in landscape mode
return landscapeLayout();
}//else( screenWidth > screenHeight) we are in landscape mode
}//********************** Widget build ends *****************************
}//*************** class _UserInstructionsState ends **********************
I have created 2 functions:-
Scaffold portraitLayout() {
return Scaffold (
body: Centre(
.
.
.
}//*********** function portraitLayout ends************
Scaffold landscapeLayout() {
return Scaffold (
body: Centre(
.
.
.
}//**************** function landscapeLayout ends *******************
these functions provide the differing screen layouts for the two orientations.
When I rotate the simulator screen between orientations I would like the new orientation layout to take effect. Only way I can achieve this at the moment is by using hot reload. I have tried using
double screenWidth = (MediaQuery.of(context).size.width) inside my functions but the compiler complains giving me a context error 'JsObject' can't be assigned to the parameter type 'BuildContext'.
I’m at a loss any help would be appreciated.
There is a Material Widget called layout builder that can help you achieve this very efficiently.
The sample code is as follows.
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
/// This is the main application widget.
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Code Sample';
#override
Widget build(BuildContext context) {
return const MaterialApp(
title: _title,
home: MyStatelessWidget(),
);
}
}
/// This is the stateless widget that the main application instantiates.
class MyStatelessWidget extends StatelessWidget {
const MyStatelessWidget({Key? key}) : super(key: key);
#override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('LayoutBuilder Example')),
body: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
if (MediaQuery.of(context).orientation ==
Orientation.portrait) {
return _buildNormalContainer();
} else {
return _buildWideContainers();
}
},
),
);
}
Widget _buildNormalContainer() {
return Center(
child: Container(
height: 100.0,
width: 100.0,
color: Colors.red,
),
);
}
Widget _buildWideContainers() {
return Center(
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Container(
height: 100.0,
width: 100.0,
color: Colors.red,
),
Container(
height: 100.0,
width: 100.0,
color: Colors.yellow,
),
],
),
);
}
}
You can find the documentation here. Documentation
There is a red customView and a button in the page:
I want to change the customView's color to green when I tap the button.
Require:
You must call customView's function changeColor to achieve it;
You can't call page's setState, it's stateless;
Do not use eventBus or provider.
Here is all my code, you can copy and test, input your code in CustomView's changeColor, I desire the easiest way.
import 'package:flutter/material.dart';
class RefreshOutsidePage extends StatelessWidget {
const RefreshOutsidePage({Key key}) : super(key: key);
#override
Widget build(BuildContext context) {
CustomView customView = CustomView();
return Scaffold(
appBar: AppBar(title: Text('refresh outside')),
body: Column(
children: [
customView,
SizedBox(height: 30),
RaisedButton(
child: Text('refresh outside'),
onPressed: () {
customView.changeColor();
},
),
],
),
);
}
}
class CustomView extends StatefulWidget {
CustomView({Key key}) : super(key: key);
#override
_CustomViewState createState() => _CustomViewState();
void changeColor() {
// input your code here
print('change');
}
}
class _CustomViewState extends State<CustomView> {
Color color = Colors.red;
void changeColor() {
setState(() {
color = Colors.green;
});
}
#override
Widget build(BuildContext context) {
return Container(
width: 200,
height: 200,
color: color,
);
}
}
You can change it through key:
Make CustomViewState public (remove _ at beginning)
Define key and call function changeColor:
class RefreshOutsidePage extends StatelessWidget {
const RefreshOutsidePage({Key key}) : super(key: key);
final _customViewKey = GlobalKey<CustomViewState>();
#override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('refresh outside')),
body: Column(
children: [
CustomView(key: _customViewKey),
SizedBox(height: 30),
RaisedButton(
child: Text('refresh outside'),
onPressed: () {
_customViewKey.currentState.changeColor();
},
),
],
),
);
}
}
There are two main solutions.
Key solution as mentioned by Autocrab.
State solution where the parent widget becomes Stateful or implement any state management solution in Flutter to update the values of his child.
The child CustomView should be Stateless as it is now because you are not changing the state within widget. So you just require extra parameters received from the parent widget to properly update or get a reference to the widget with the GlobalKey to update it.
If you are using this project for learning or something not legacy I suggest you upgrade Flutter as RaisedButton is deprecated and on the long term you will also have to use null-safety. But that is outside the scope of this question.
Shortly, I need the selectable area to be smaller in terms of height.
Text and Checkbox sizes are good, but the surrounding box is too big for the checklist I'm trying to create.
CheckBoxListTile current height vs. desired height
Tried wrapping it with Transform.scale but text becomes too small:
Transform.scale(
scale: 0.8,
child: CheckboxListTile(
title: const Text("Rinite alérgica"),
value: timeDilation != 1.0,
controlAffinity: ListTileControlAffinity.leading,
onChanged: (bool value) {
setState(() {
timeDilation = value ? 2.0 : 1.0;
});
},
),
),
Tried wrapping it with Container, but I get on-screen overflow warnings.
Does anyone have a better solution?
When trying to resize the CheckboxListFile it seems that Google actually recommends creating a custom Tile widget and making use of the Checkbox widget.
https://api.flutter.dev/flutter/material/CheckboxListTile-class.html#material.CheckboxListTile.3
Look at the LabeledCheckbox widget that's been created. You can very easily modify all components to fit your needs. For example, if you wished to make the Widget itself smaller, you can now wrap it in a Container
/// Flutter code sample for CheckboxListTile
// 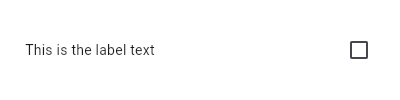
//
// Here is an example of a custom LabeledCheckbox widget, but you can easily
// make your own configurable widget.
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
/// This is the main application widget.
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Code Sample';
#override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: const Center(
child: MyStatefulWidget(),
),
),
);
}
}
class LabeledCheckbox extends StatelessWidget {
const LabeledCheckbox({
Key key,
#required this.label,
#required this.padding,
#required this.value,
#required this.onChanged,
}) : super(key: key);
final String label;
final EdgeInsets padding;
final bool value;
final Function onChanged;
#override
Widget build(BuildContext context) {
return InkWell(
onTap: () {
onChanged(!value);
},
child: Container(
padding: padding,
child: Row(
children: <Widget>[
Expanded(child: Text(label)),
Checkbox(
value: value,
onChanged: (bool newValue) {
onChanged(newValue);
},
),
],
),
),
);
}
}
/// This is the stateful widget that the main application instantiates.
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({Key? key}) : super(key: key);
#override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
/// This is the private State class that goes with MyStatefulWidget.
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool _isSelected = false;
#override
Widget build(BuildContext context) {
return LabeledCheckbox(
label: 'This is the label text',
padding: const EdgeInsets.symmetric(horizontal: 20.0),
value: _isSelected,
onChanged: (bool newValue) {
setState(() {
_isSelected = newValue;
});
},
);
}
}
Have you tried ?
SizedBox(
height: 50,
width: 50,
child: ......
)