While coding an app i realized, that if you use a hint: with the DropdownButton and a value you only see the value. After some research and trying to work my way around it i finally found a solution. Idk if this is helpful or not but i wanted to share this with you and maybe it does help you in your own project. But without further ado here is the "not functional code":
import 'package:flutter/material.dart';
void main() => runApp(const ButtonClass());
class ButtonClass extends StatefulWidget {
const ButtonClass({Key? key}) : super(key: key);
#override
State<ButtonClass> createState() => _ButtonClassState();
}
class _ButtonClassState extends State<ButtonClass> {
List<DropdownMenuItem<String>> get dropdownItems {
List<DropdownMenuItem<String>> menuItems = [
const DropdownMenuItem(child: Text("One"), value: "Option1"),
const DropdownMenuItem(child: Text("Two"), value: "Option2"),
const DropdownMenuItem(
child: Text("Three"),
value: "Option3",
),
const DropdownMenuItem(
child: Text("Four"),
value: "Option4",
),
const DropdownMenuItem(
child: Text("Five"),
value: "Option5",
),
];
return menuItems;
}
String selectedValue = "Option1";
#override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(
child: Container(
width: 200.0,
height: 200.0,
child: DropdownButtonHideUnderline(
child: DropdownButton(
isExpanded: true,
hint: const Center(
child: FittedBox(
fit: BoxFit.contain,
child: Text(
"Displayed Text",
style: TextStyle(
color: Colors.black,
fontSize: 30.0,
fontFamily: 'Arial',
),
),
),
),
items: dropdownItems,
value: selectedValue,
onChanged: (String? newValue) {
setState(() {
selectedValue = newValue!;
});
},
),
),
),
),
),
);
}
}
And here is the solution:
Change the
String selectedValue = "Option1";
to (example)
String? _selectedColor;
and also change
value: selectedValue,
onChanged: (String? newValue) {
setState(() {
selectedValue = newValue!;
});
},
to
value: _selectedColor,
onChanged: (String? newValue) {
setState(() {
_selectedColor= newValue!;
});
},
Here is the full main.dart file:
import 'package:flutter/material.dart';
void main() => runApp(const ButtonClass());
class ButtonClass extends StatefulWidget {
const ButtonClass({Key? key}) : super(key: key);
#override
State<ButtonClass> createState() => _ButtonClassState();
}
class _ButtonClassState extends State<ButtonClass> {
List<DropdownMenuItem<String>> get dropdownItems {
List<DropdownMenuItem<String>> menuItems = [
const DropdownMenuItem(child: Text("One"), value: "Option1"),
const DropdownMenuItem(child: Text("Two"), value: "Option2"),
const DropdownMenuItem(
child: Text("Three"),
value: "Option3",
),
const DropdownMenuItem(
child: Text("Four"),
value: "Option4",
),
const DropdownMenuItem(
child: Text("Five"),
value: "Option5",
),
];
return menuItems;
}
String? _selectedColor;
#override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(
child: Container(
width: 200.0,
height: 200.0,
child: DropdownButtonHideUnderline(
child: DropdownButton(
isExpanded: true,
hint: const Center(
child: FittedBox(
fit: BoxFit.contain,
child: Text(
"Displayed Text",
style: TextStyle(
color: Colors.black,
fontSize: 30.0,
fontFamily: 'Arial',
),
),
),
),
items: dropdownItems,
value: _selectedColor,
onChanged: (String? newValue) {
setState(() {
_selectedColor = newValue!;
});
},
),
),
),
),
),
);
}
}
Related
I have a DropdownButton which displays user type.
List<String> items = ['Engineer', 'Technician', 'Sales'];
String? currentSelectedValue;
child: DropdownButtonHideUnderline(
child: Padding(
padding:
const EdgeInsets.symmetric(horizontal: 20.0),
child: DropdownButton<String>(
dropdownColor: Colors.blue.shade100,
isExpanded: true,
hint: Text('Select the user Type'),
onChanged: (newValue) {
setState(() {
currentSelectedValue = newValue;
});
print(currentSelectedValue);
},
items: items.map((String value) {
return DropdownMenuItem(
value: value,
child: Text(
value,
style: TextStyle(color: Colors.black),
),
);
}).toList(),
value: currentSelectedValue,
),
),
),
I can see the list, but when I select a value, it is not displaying on the Text portion of the DropdownButton. I could see the selected value printed in the console.
Can anyone help me to find the mistake?
Make sure to put currentSelectedValue outside the build method.
class Ft extends StatefulWidget {
const Ft({super.key});
#override
State<Ft> createState() => _FtState();
}
class _FtState extends State<Ft> {
List<String> items = ['Engineer', 'Technician', 'Sales'];
String? currentSelectedValue;
#override
Widget build(BuildContext context) {
return Scaffold(
body: DropdownButtonHideUnderline(
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 20.0),
child: DropdownButton<String>(
dropdownColor: Colors.blue.shade100,
isExpanded: true,
hint: Text('Select the user Type'),
onChanged: (newValue) {
setState(() {
currentSelectedValue = newValue;
});
print(currentSelectedValue);
},
items: items.map((String value) {
return DropdownMenuItem(
value: value,
child: Text(
value,
style: TextStyle(color: Colors.black),
),
);
}).toList(),
value: currentSelectedValue,
),
),
),
);
}
}
I have tried to build a dropdown button and a menu with it, where the value will be selected from the dropdown menu. The code is as below:
String valueChoose;
List listItem = ["A", "B", "C", "D", "E"];
DropdownButton(
hint: Text('Associate'),
dropdownColor: Colors.white,
icon: Icon(Icons.arrow_drop_down),
iconSize: 20.0,
style: TextStyle(
fontSize: 22.0,
color: Colors.black,
),
value: valueChoose,
onChanged: (newValue) {
setState(() {
valueChoose = newValue;
});
},
items: listItem.map((valueItem){
return DropdownMenuItem(
value: valueItem,
child: Text(valueItem),
);
}).toList(),
),
The error I'm facing is in the set state, where I've assigned newValue to the valueChoose.
A value of type 'Object?' can't be assigned to a variable of type 'String'.
Try changing the type of the variable, or casting the right-hand type to 'String'.
That is the error showing up for the newValue assinged in the set state. Please help regarding this, thanks in advance!
Below is the code, including the AlertDailog:
class HomeScreen extends StatefulWidget {
#override
_HomeScreenState createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
String ? valueChoose;
List listItem = [
"A", "B", "C", "D", "E"
];
void assignPopup(BuildContext context) {
var alertDialog = AlertDialog(
content:
Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Row(
children:[
Container(
child: Text(
'Action',
),
),
]
),
Row(
children:[
Container(
child: Card(
elevation: 5.0,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10.0),
),
child: TextField(
decoration: InputDecoration(
labelText: 'Please add any comments',
),
),
),
),
]
),
Row(
children:[
Container(
child: Text(
'Assign To',
),
),
]
),
Row(
children: [
Container(
child: Card(
elevation: 5.0,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10.0),
),
child: DropdownButton<String>(
hint: Text('Associate'),
dropdownColor: Colors.white,
icon: Icon(Icons.arrow_drop_down),
iconSize: 40.0,
style: TextStyle(
fontSize: 18.0,
color: Colors.black,
),
value: valueChoose,
onChanged: (newValue) {
setState(() {
valueChoose = newValue;
});
},
items: listItem.map<DropdownMenuItem<String>>((valueItem){
return DropdownMenuItem(
value: valueItem,
child: Text(valueItem),
);
}).toList(),
),
),
),
],
),
],
),
);
showDialog(
context: context,
builder: (BuildContext context) {
return alertDialog;
}
);
}
#override
Widget build(BuildContext context) {
return Scaffold(
...
...
Container(
child: TextButton(
onPressed: (){
assignPopup(context);
},
child: Text(
'Assign',
),
),
),
);
}
}
From the data that you provided I have created a example where I have used the alertdialog and inside it there is a drop down
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
#override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: HomeScreen(),
);
}
}
class HomeScreen extends StatefulWidget {
#override
_HomeScreenState createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
String valueChoose;
List listItem = ["A", "B", "C", "D", "E"];
void assignPopup(BuildContext context) {
showDialog(
context: context,
builder: (BuildContext context) {
return StatefulBuilder(builder: (context, setState) {
return AlertDialog(
content: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Row(children: [
Container(
child: Text(
'Action',
),
),
]),
Row(children: [
Expanded(
child: Container(
child: Card(
elevation: 5.0,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10.0),
),
child: TextField(
decoration: InputDecoration(
labelText: 'Please add any comments',
),
),
),
),
),
]),
Row(children: [
Container(
child: Text(
'Assign To',
),
),
]),
Row(
children: [
Container(
child: Card(
elevation: 5.0,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10.0),
),
child: DropdownButton<String>(
hint: Text('Associate'),
dropdownColor: Colors.white,
icon: Icon(Icons.arrow_drop_down),
iconSize: 40.0,
style: TextStyle(
fontSize: 18.0,
color: Colors.black,
),
value: valueChoose,
onChanged: (newValue) {
setState(() {
valueChoose = newValue;
});
},
items: listItem
.map<DropdownMenuItem<String>>((valueItem) {
return DropdownMenuItem(
value: valueItem,
child: Text(valueItem),
);
}).toList(),
),
),
),
],
),
],
),
);
});
});
}
#override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Container(
child: TextButton(
onPressed: () {
assignPopup(context);
},
child: Text(
'Assign',
),
),
),
),
);
}
}
So here in order to change dropdown value you have to use the StatefulBuilder which will change your dropdown value. you can check the above example and make changes as per your needs.
Please run the code to check the desired output.
Let me know if it works.
Specify the types of DropdownButton and that map
String? valueChoose;
List listItem = ["A", "B", "C", "D", "E"];
DropdownButton<String>(
hint: Text('Associate'),
dropdownColor: Colors.white,
icon: Icon(Icons.arrow_drop_down),
iconSize: 20.0,
style: TextStyle(
fontSize: 22.0,
color: Colors.black,
),
value: valueChoose,
onChanged: (newValue) {
setState(() {
valueChoose = newValue;
});
},
items: listItem.map<DropdownMenuItem<String>>((valueItem) {
return DropdownMenuItem(
value: valueItem,
child: Text(valueItem),
);
}).toList(),
)
Declare variables outside the build method.
String? valueChoose;
List listItem = ["A", "B", "C", "D", "E"];
Inside the build method add the Dropdown widget, So the complete state class code would look like this,
class _DropdownViewState extends State<DropdownView> {
String? valueChoose;
List listItem = ["A", "B", "C", "D", "E"];
#override
Widget build(BuildContext context) {
return DropdownButton<String>(
hint: Text('Associate'),
dropdownColor: Colors.white,
icon: Icon(Icons.arrow_drop_down),
iconSize: 20.0,
style: TextStyle(
fontSize: 22.0,
color: Colors.black,
),
value: valueChoose,
onChanged: (String? newValue) {
setState(() {
valueChoose = newValue;
});
},
items: listItem.map<DropdownMenuItem<String>>((valueItem){
return DropdownMenuItem(
value: valueItem,
child: Text(valueItem),
);
}).toList(),
);
}
I have multiple line items in the dropdown menu in flutter like this :
It is shown perfectly fine in the dropdown pop up but in dropdown button it shows bottomoverflow like this :
Here is code for the same :
DropdownButtonHideUnderline(
child: DropdownButton(
items: addresses.map((String value) {
return new DropdownMenuItem<String>(
value: value,
child: SizedBox(
height: 10 * SizeConfig.heightMultiplier,
child: Column(
children: [
SizedBox(height: 1.5 * SizeConfig.heightMultiplier),
new Text(value, overflow: TextOverflow.clip),
SizedBox(height: 1.5 * SizeConfig.heightMultiplier),
],
),
),
);
}).toList(),
onChanged: (String newValue) {
setState(() {
_selectedShippingAddress = newValue;
});
},
hint: Text("Select address"),
selectedItemBuilder: (BuildContext context) {
return addresses.map<Widget>((String item) {
return Container(
child: Text(item),
);
}).toList();
},
style: TextStyle(
fontSize: 1.9 *
SizeConfig.textMultiplier,
color:
Theme.of(context).accentColor,
fontFamily: 'Montserrat'),
value: _selectedShippingAddress,
isExpanded: true,
underline: Container(
height: 1,
color: Theme.of(context)
.textSelectionColor,
),
icon: Icon(Icons.arrow_drop_down),
isDense: true,
),
)
So what is a solution for this? Can anyone help on this?
From the example that you provided I replicated it and there is a problem in the blow code
DropdownMenuItem<String>(
value: value,
child: SizedBox(
height: 10 * SizeConfig.heightMultiplier,
Maybe the height is doing some problem just added a sample example below to let you know the things work.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
#override
Widget build(BuildContext context) {
return MaterialApp(
home: SampleApp(),
debugShowCheckedModeBanner: false,
);
}
}
class SampleApp extends StatefulWidget {
#override
_SampleAppState createState() => _SampleAppState();
}
class _SampleAppState extends State<SampleApp> {
String _selectedShippingAddress;
List addresses = ['sample1', 'sample 2'];
#override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Your heading'),
),
body: DropdownButtonHideUnderline(
child: DropdownButton<String>(
items: addresses.map((value) {
return new DropdownMenuItem<String>(
value: value,
child: SizedBox(
child: Column(
children: [
SizedBox(height: 5),
new Text(value, overflow: TextOverflow.clip),
SizedBox(height: 5),
],
),
),
);
}).toList(),
onChanged: (String newValue) {
setState(() {
_selectedShippingAddress = newValue;
});
},
hint: Text("Select address"),
selectedItemBuilder: (BuildContext context) {
return addresses.map<Widget>((item) {
return Container(
child: Text(item),
);
}).toList();
},
style: TextStyle(
fontSize: 14,
color: Theme.of(context).accentColor,
fontFamily: 'Montserrat'),
value: _selectedShippingAddress,
isExpanded: true,
underline: Container(
height: 1,
color: Theme.of(context).textSelectionColor,
),
icon: Icon(Icons.arrow_drop_down),
isDense: true,
),
));
}
}
Hi i got stuck while write flutter code on dropdown button, where after user choosed from the list the hint wont changed to what the user choose. Can anyone help ?
So here is my code:
DropdownButton(items: [
DropdownMenuItem(value: "1", child: Text('+')),
DropdownMenuItem(value: "2", child: Text('-')),
DropdownMenuItem(value: "3", child: Text('X')),
DropdownMenuItem(value: "4", child: Text('/'))
].toList(), onChanged: (value){
setState(() {
_value = value;
});
},hint: Text('Operation'),)
I have just created an example below just check it and let me know if it works :
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
#override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
#override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
String selectedOperator;
var listOfOperators = [
Operators(type: "+ Addition", value: 1),
Operators(type: "- Substraction", value: 2),
Operators(type: "* Multiplication", value: 3),
Operators(type: "/ Division", value: 4),
];
#override
void initState() {
super.initState();
print(listOfOperators.length);
}
#override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
body: Center(
child: Container(
child: Padding(
padding: const EdgeInsets.all(30.0),
child: Container(
height: 50,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(5.0),
border: Border.all(
color: Colors.red, style: BorderStyle.solid, width: 0.80),
),
child: DropdownButton(
value: selectedOperator,
isExpanded: true,
icon: Padding(
padding: const EdgeInsets.only(left: 15.0),
child: Icon(Icons.arrow_drop_down),
),
iconSize: 25,
underline: SizedBox(),
onChanged: (newValue) {
setState(() {
print(newValue);
selectedOperator = newValue;
});
print(selectedOperator);
},
hint: Padding(
padding: const EdgeInsets.all(8.0),
child: Text('Select'),
),
items: listOfOperators.map((data) {
return DropdownMenuItem(
value: data.value.toString(),
child: Padding(
padding: const EdgeInsets.only(left: 10.0),
child: Text(
data.type,
style: TextStyle(
fontSize: 18,
color: Colors.black,
),
),
),
);
}).toList()),
),
),
),
),
),
);
}
}
class Operators {
String type;
int value;
Operators({this.type, this.value});
}
Here you go with running example:
String dropdownValue = 'Lahore';
#override
Widget build(BuildContext context) {
return DropdownButton<String>(
value: dropdownValue,
icon: Icon(Icons.arrow_downward),
iconSize: 24,
elevation: 16,
style: TextStyle(
color: Colors.deepPurple
),
underline: Container(
height: 2,
color: Colors.deepPurpleAccent,
),
onChanged: (String newValue) {
setState(() {
dropdownValue = newValue;
});
},
items: <String>['Lahore', 'Islamabad', 'Faisalabad', 'Attabad']
.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
})
.toList(),
);
}
I am using the RadioListTile for displaying the dynamic data with radio button in flutter,but I want to display the same list with an trailing icon for editing the list data.
Can you please help me how to do that in flutter?
RadioListTile(
groupValue: _currentIndex,
title: Text(
// addres["address_1"],
addres["firstname"] +
addres["lastname"] +
addres["address_1"] +
addres["city"] +
addres["country"],
overflow: TextOverflow.ellipsis,
maxLines: 3,
style: TextStyle(color: Colors.black, fontSize: 16.0),
),
value: int.parse(addres["address_id"]),
onChanged: (val) {
setState(() {
_currentIndex = val;
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => PickanAddresspage(selected_address: addres['address_id'],)));
});
},
)
In title , you can user Row to wrap Text and Icon
Use Expanded and flex to control width you need
code snippet
title: Row(
children: <Widget>[
Expanded(
flex: 3,
child: Text(
"firstname" +
"lastname" +
"address_1" +
"city" +
"country234123412344523523452542345234523523542345245234523452345235234523452345245234523452452542",
overflow: TextOverflow.ellipsis,
maxLines: 3,
style: TextStyle(color: Colors.black, fontSize: 16.0),
),
),
Expanded(
flex: 1,
child: InkWell(
child: Icon(
Icons.audiotrack,
color: Colors.green,
size: 30.0,
),
),
)
],
)
full test code
// Flutter code sample for
// 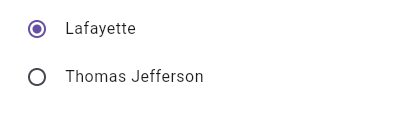
//
// This widget shows a pair of radio buttons that control the `_character`
// field. The field is of the type `SingingCharacter`, an enum.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
/// This Widget is the main application widget.
class MyApp extends StatelessWidget {
static const String _title = 'Flutter Code Sample';
#override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: MyStatefulWidget(),
),
);
}
}
enum SingingCharacter { lafayette, jefferson }
class MyStatefulWidget extends StatefulWidget {
MyStatefulWidget({Key key}) : super(key: key);
#override
_MyStatefulWidgetState createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
SingingCharacter _character = SingingCharacter.lafayette;
#override
Widget build(BuildContext context) {
return Column(
children: <Widget>[
RadioListTile<SingingCharacter>(
title: const Text('Lafayette'),
value: SingingCharacter.lafayette,
groupValue: _character,
onChanged: (SingingCharacter value) {
setState(() {
_character = value;
});
},
),
RadioListTile<SingingCharacter>(
title: Row(
children: <Widget>[
Expanded(
flex: 3,
child: Text(
"firstname" +
"lastname" +
"address_1" +
"city" +
"country234123412344523523452542345234523523542345245234523452345235234523452345245234523452452542",
overflow: TextOverflow.ellipsis,
maxLines: 3,
style: TextStyle(color: Colors.black, fontSize: 16.0),
),
),
Expanded(
flex: 1,
child: InkWell(
child: Icon(
Icons.audiotrack,
color: Colors.green,
size: 30.0,
),
),
)
],
),
value: SingingCharacter.jefferson,
groupValue: _character,
onChanged: (SingingCharacter value) {
setState(() {
_character = value;
});
},
),
],
);
}
}